Cron triggers
Trigger jobs based on a Cron schedule.
Creating Cron tasks
Cron tasks run repeatedly on a specified cron schedule.
To create a Cron task, use tilebox.workflows.automations.CronTask
as your tasks base class instead of the regular tilebox.workflows.Task
.
Registering a Cron trigger
After implementing a Cron task, register it to be triggered according to a Cron schedule. When the Cron expression matches, a new job is submitted consisting of a single task instance derived from the Cron task prototype.
The syntax for specifying the cron triggers is a CRON expression. A helpful tool to test your cron expressions is crontab.guru.
Starting a Cron Task Runner
With the Cron automation registered, a job is submitted whenever the Cron expression matches. But unless a task runner is available to execute the Cron task the submitted jobs remain in a task queue. Once an eligible task runner becomes available, all jobs in the queue are executed.
If this task runner runs continuously, its output may resemble the following:
Inspecting in the Console
The Tilebox Console provides a straightforward way to inspect all registered Cron automations.
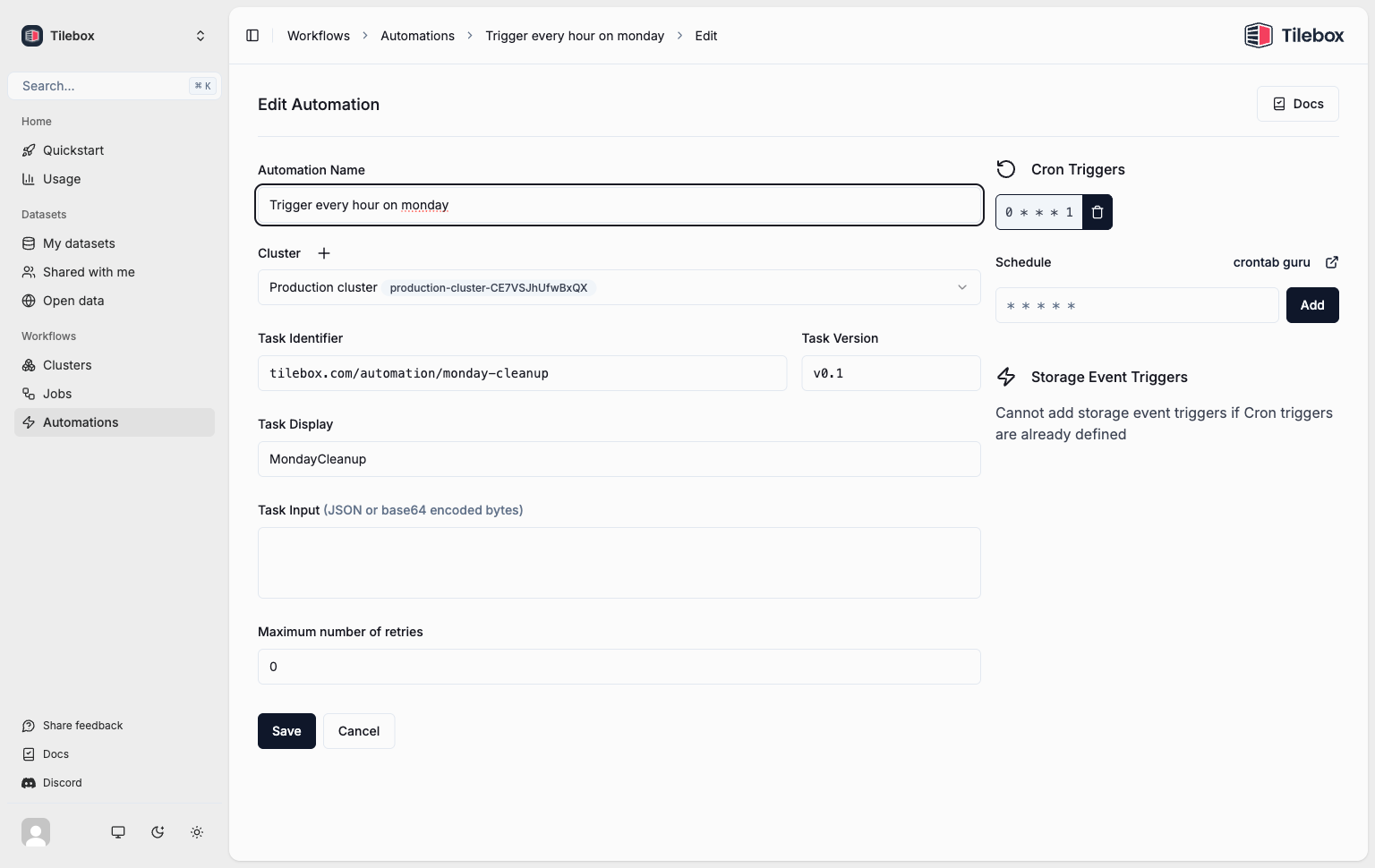

Use the console to view, edit, and delete the registered Cron automations.
Deleting Cron automations
To delete a registered Cron automation, use automations.delete
. After deletion, no new jobs will be submitted by that Cron trigger. Past jobs already triggered will still remain queued.
Submitting Cron jobs manually
You can submit Cron tasks as regular tasks for testing purposes or as part of a larger workflow. To do so, instantiate the task with a specific trigger time using the once
method.
Submitting a job with a Cron task using once
immediately schedules the task, and a runner may pick it up and execute it. The trigger time set in the once
method does not influence the execution time; it only sets the self.trigger.time
attribute for the Cron task.
Was this page helpful?